Mapping F12 to Play/Pause on a Lenovo Laptop in Windows 10 using Lenovo Vantage
Lenovo laptops have many functions mapped to the function keys on the top row, but (at least on my laptop) media controls are not among them.
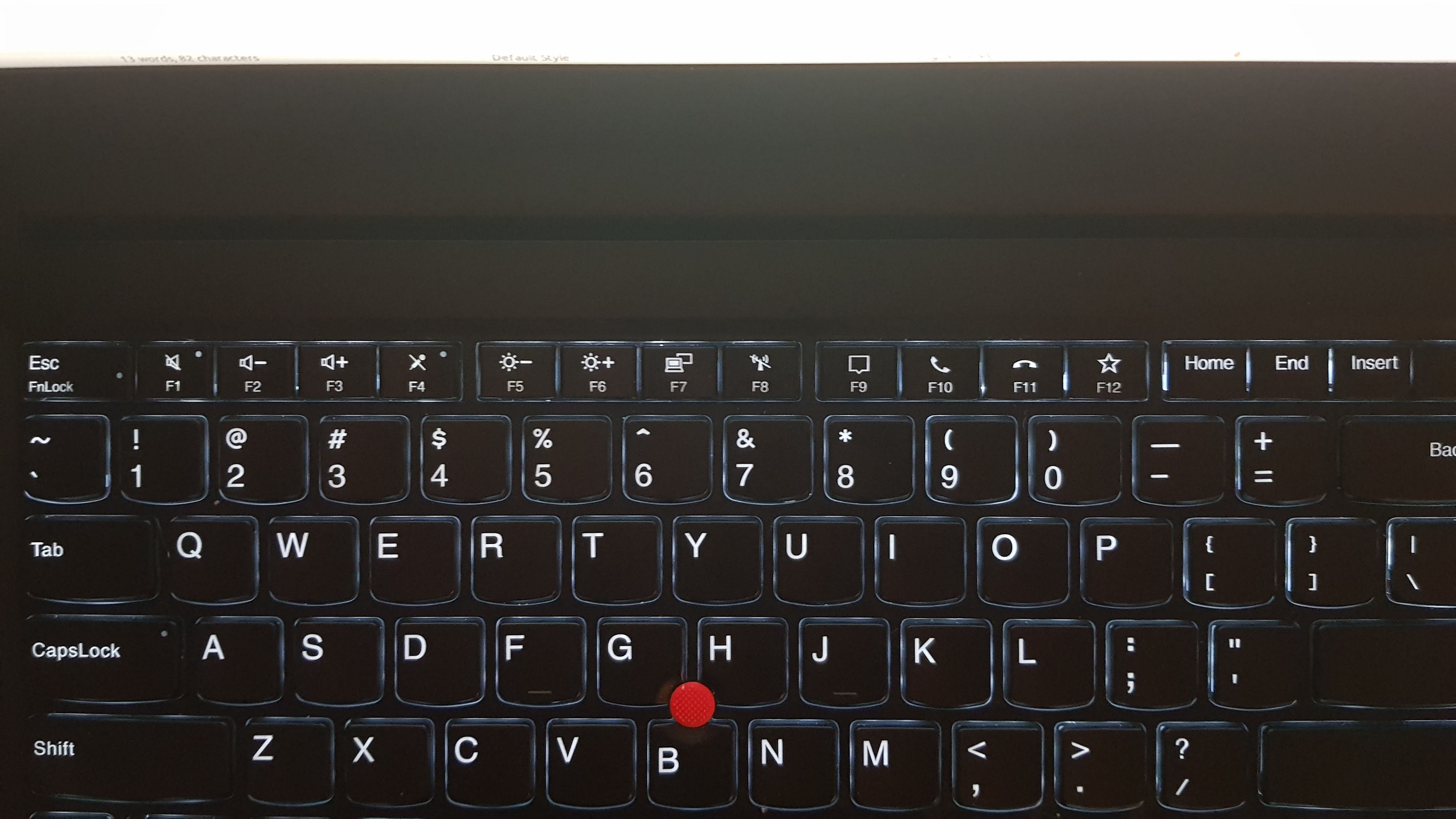
Media controls (play/pause and next/previous) are notably absent from my Lenovo laptop keyboard
Lenovo laptops have a “User-defined Key” on F12 (may be different on your laptop, it is the key with the star on it) which can be mapped to a limited number of functions through the Lenovo Vantage application. Although media controls are not among the selectable functions, there is one option that we can use to our advantage: Open applications or files. The “Open applications or files” option for the User-defined key allows us to perform custom actions
We are going to create a simple C# application that triggers a virtual keyboard event for the media play/pause button when the application is open and then configure Lenovo Vantage to open it when the F12 key is pressed. This allows us to have a play/pause button on our keyboard.
In your plain text editor of choice (I used Notepad++) create a file called f12-play-pause.cs
; this will be the source code for our application. In it, add the following text:
using System;
using System.Runtime.InteropServices;
public class Program
{
public const int KEYEVENTF_EXTENDEDKEY = 1;
public const int KEYEVENTF_KEYUP = 0;
public const int VK_MEDIA_PLAY_PAUSE = 0xB3;
[DllImport("user32.dll")]
public static extern void keybd_event(byte virtualKey, byte scanCode, uint flags, IntPtr extraInfo);
public static void Main()
{
keybd_event(VK_MEDIA_PLAY_PAUSE, KEYEVENTF_KEYUP, KEYEVENTF_EXTENDEDKEY, IntPtr.Zero);
}
}
When this program is run, it will execute the Main()
method, which has one command: trigger a keyboard event for the MEDIA_PLAY_PAUSE
key. Once this single command is run, the application is closed and you are returned to what you were doing before. In practice, this happens so quickly that there is virtually no interruption and the only indication that an application is being opened for this is a slight flicker in the Taskbar.
Now that we have the source code for our application, we need to compile it into an exe file. If you do not already have a C# development environment set up, the easiest way I have found to compile this application is to use CS-Script.
In order to compile the script using CS-Script you first need to install it; this can be easily done by downloading the latest release from Github, extracting the folder (you might also need to install 7-Zip to open the archive), and double-clicking install.cmd
. Once we are done it can be easily uninstalled by double-clicking uninstall.cmd
if you will not be needing it again.
Now that we have CS-Script installed, we can open a cmd window and navigate to the folder where we saved f12-play-pause.cs
. First test that your script is working correctly by running:
cscs f12-play-pause.cs
Once you are sure that the script runs correctly using this command, run the following command to compile it to a file (-e
stands for export, in that it is exporting the compiled application to a file instead of just running it):
cscs -e f12-play-pause.cs
This will generate a new file called f12-play-pause.exe
, which should simulate a play/pause button press when opened. Make sure this application is working properly and move it to a place that you are okay with keeping it permanently.
If you used a method other than CS-Script to compile our application, such as Visual Studio, you may need to change the output type from Console Application to Windows Application to avoid having a cmd window flicker into existance while the application runs.
Finally, it is time to connect our new application to the F12/User-defined key. Open Lenovo Vantage and select “Input & accessories” from the Device dropdown. The dropdown where the User-defined Key settings are found in Lenovo Vantage
Scroll down and select the “Open applications or files” option in the Action User Defined Key dropdown. You will now be presented with two options: Applications to open and Files to open. Interestingly, our new application is not available through the Applications option, so we need to instead click on the Add button in the “Files to open” section and select our f12-play-pause.exe
application.
Our application will not display in the list unless you restart Lenovo Vantage (after which it will appear as a nameless entry in the Applications list), but now when you click F12 on your keyboard your music will toggle between play and pause.
I am, of course, always looking for ways to improve this setup; feel free to add any comments, questions, or suggestions you might have in the comments section below.